Okay, sketch 2: Boids!
The basic idea is to create a bunch of particles (the Boids in this case) and apply to them each a series of simple, limited rules that rely neither on communcation between the Boids nor a global controller and see what behaviors you can generate. Specifically, can you replicate the flocking behavior found in birds, since birds can obviously fly together without hitting one another and also without some lead bird giving orders.
Something like this:
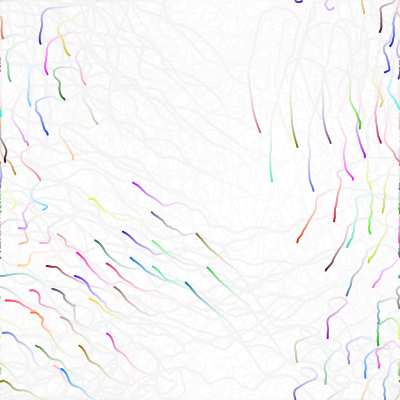
For this case, there are three rules:
- seperation - Fly away from any Boids that are too close to you (to avoid collision)
- alignment - Align yourself to fly in the same direction as any Boids in your field of vision
- cohesion - Fly towards the center point of the Boids you can see