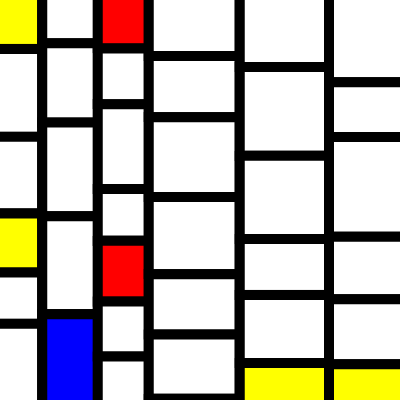
Spend a month making one beautiful thing per day, given a bunch of prompts. A month late, but as they say, ’the second best time is now'.
Let’s do it!
30) Minimalism
let gui;
let params = {
lineWidth: 10,
minWidth: 50,
maxWidth: 100, maxWidthMax: 400,
minHeight: 50,
maxHeight: 100, maxHeightMax: 400,
colorChance: 0.1, colorChanceMin: 0, colorChanceMax: 1, colorChanceStep: 0.01,
}
let box;
let colors = [
"red",
"blue",
"yellow",
]
function setup() {
createCanvas(400, 400);
box = {
x: -params.lineWidth,
y: -params.lineWidth,
width: random(params.minWidth, params.maxWidth),
height: random(params.minHeight, params.maxHeight),
}
gui = createGuiPanel('params');
gui.addObject(params);
gui.setPosition(420, 0);
}
function draw() {
strokeWeight(params.lineWidth);
stroke("black");
if (random() < params.colorChance) {
fill(random(colors));
} else {
fill("white");
}
rect(box.x, box.y, box.width, box.height);
box.y += box.height;
box.height = random(params.minHeight, params.maxHeight);
if (box.y > height) {
box.x += box.width;
box.y = -params.lineWidth;
box.width = random(params.minWidth, params.maxWidth);
box.height = random(params.minHeight, params.maxHeight);
}
if (box.x > width) {
noLoop();
}
}
I did have a sketch before that that ended up getting a little un-minimal:
let gui;
let params = {
cycle: 10,
refresh: 10,
offset: 20,
radius: 100,
}
let x, y;
function setup() {
createCanvas(400, 400);
x = random(200) + 100;
y = random(200) + 100;
gui = createGuiPanel('params');
gui.addObject(params);
gui.setPosition(420, 0);
}
function draw() {
stroke("black");
noFill();
if (frameCount % (params.cycle * params.refresh) == 0) {
background("white");
}
if (frameCount % params.cycle == 0) {
x = random(200) + 100;
y = random(200) + 100;
}
circle(
x + params.offset * cos(frameCount / params.cycle),
y + params.offset * sin(frameCount / params.cycle),
params.radius,
);
}
It’s just circles! But yeah.
Posts in Genuary 2023:
- Genuary 2023.01: Perfect loop
- Genuary 2023.02: Made in 10 minutes
- Genuary 2023.03: Glitch art
- Genuary 2023.04: Intersections
- Genuary 2023.05: Debug view
- Genuary 2023.06: Steal like an artist
- Genuary 2023.07: Sample a color palette
- Genuary 2023.08: Signed Distance Functions
- Genuary 2023.09: Plants
- Genuary 2023.10: Generative Music
- Genuary 2023.11: Suprematism
- Genuary 2023.12: Tessellation
- Genuary 2023.13: Something you've always wanted to learn
- Genuary 2023.14: Asemic Writing
- Genuary 2023.15: Sine Waves
- Genuary 2023.16: Reflections of a Reflection
- Genuary 2023.17: A grid inside a grid inside a grid
- Genuary 2023.18: Definitely not a grid
- Genuary 2023.19: Black and white
- Genuary 2023.20: Art Deco
- Genuary 2023.21: Persian Carpet
- Genuary 2023.22: Shadows
- Genuary 2023.23: Moiré
- Genuary 2023.24: Textile
- Genuary 2023.25: Yayoi Kusama
- Genuary 2023.26: My kid could have made that
- Genuary 2023.27: In the style of Hilma Af Klint
- Genuary 2023.28: Generative poetry
- Genuary 2023.29: Maximalism
- Genuary 2023.30: Minimalism
- Genuary 2023.31: Break a previous image