I had a script that would take a file and a passphrase and either encrypt it or, if already encrypted, decrypt it. It worked well enough and I got to play with the struct
library. But it was home grown–so not compatible with anything–and didn’t properly validate anything. It worked well enough, but perhaps I could do something better.
Enter aes 2.0.
This time around, it’s just a thin wrapper around OpenSSL, originally based on the commands in this article. To encrypt a file:
dst=$src.aes
openssl enc -e -aes256 -in $src -out $dst && rm $src
To decrypt:
dst=${src::${#src}-4}
openssl enc -d -aes256 -in $src -out $dst && rm $src || (rm $dst; false)
And wrap that all up with a bit of magical filenames to do the right thing(bur for real):
for src in "$@"
do
if [[ "$src" == *.aes ]]
then
echo "Decrypting $src"
dst=${src::${#src}-4}
openssl enc -d -aes256 -in $src -out $dst && rm $src || (rm $dst; false)
else
echo "Encrypting $src"
dst=$src.aes
openssl enc -e -aes256 -in $src -out $dst && rm $src
fi
done
And it works great:
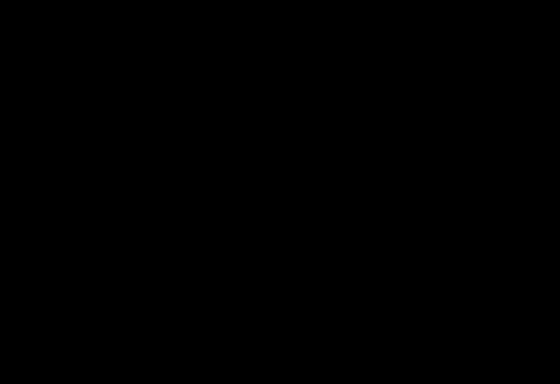
Excellent.
But wait…
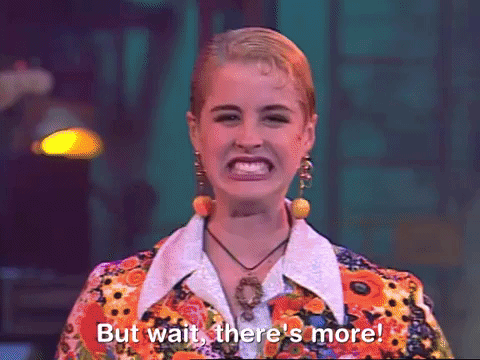
There’s more.
if [[ "$src" == *.aesdir ]]
then
echo "Decrypting directory $src"
dst=${src::${#src}-7}
(openssl enc -d -aes256 -in $src | tar xf -) && rm $src
elif [[ -d "$src" ]]
then
echo "Encrypting directory $src"
dst=$src.aesdir
(tar -czf - $src | openssl enc -e -aes256 -out $dst) && rm -rf $src
...
Let’s do directories! Basically, if it’s given a directory instead of a file, tar it up then encrypt it (with a different extention). If you see that extension, decrypt it and untar it.
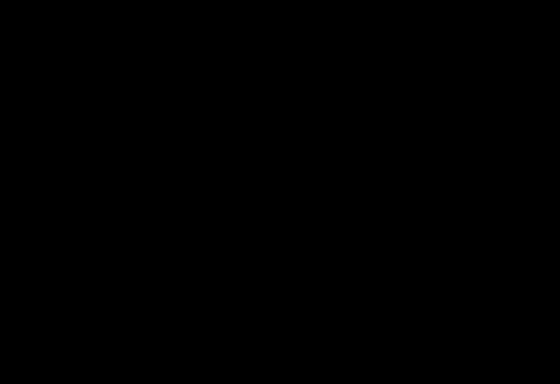
Recordings generated via Terminalizer with a wrapper. I’ll write that up soon(tm).
All in all, it’s pretty handy. I’ll have to move my files over at some point, but for now, onwards!